Quest
Modeling games using OOP
This lab builds on the the Uno Lab, where you learned about Object-Oriented programming. OOP solves problems by creating models of situations, with an object representing each person or thing in the situation. In this lab, you will learn how to use a framwork called Quest to make video games. Specifically, Quest is a framework for making top-down role-playing games where you control a character and explore a world. The Legend of Zelda (1986) was one of the original top-down role-playing games, and it continues to have a big impact on the style of games today.
Look at the GIF above from the original Zelda game, and think about the classes that you would need to make this game. Probably something like:
- Game to keep track of everything else
- Map to show the map
- Terrain to represent the bushes and stuff
- Monster for those spiky creatures with tongues
- Character That’s you!
- Infobar for the black bar at the top
- Minimap for the small map
- Stats for how much life you have left, etc.
- Map to show the map
This would take a ton of work! Quest is a tool kit (also called a framework) containing commonly-needed classes for this kind of game. Each class works in a default way, and then you can customize it to work just the way you want it to. Using tool kits like this can help you write powerful programs.
The down side is that you are working with lots and lots of code that you didn’t write. In fact, Quest is built using another framework called Arcade for making games of all kinds. Arcade is built on top of a framework called Pyglet for making desktop apps which use the keyboard and mouse, and Pyglet depends on many more layers of lower-level stuff. This can quickly get overwhelming, which is why we rely on abstraction. Quest is limited, but it’s simple. Its goal is to let you make a certain kind of game without having to learn about all the underlying parts.
Reading documentation
Now that you’re working with lots of code written by other people, you need to learn how to read documentation. Here’s a link to the documentation for Quest; keep this open in a new tab.
👀 In fact, before you go any further, have a quick skim over the Quest documentation. The Reference section provides a list of all the classes in Quest. All the contributed modules were written by Making With Code students in previous years!
Let’s play some games
💻
From a poetry shell, run python island.py
.
You should find yourself exploring an island. Close the window when you’re
tired of exploring.
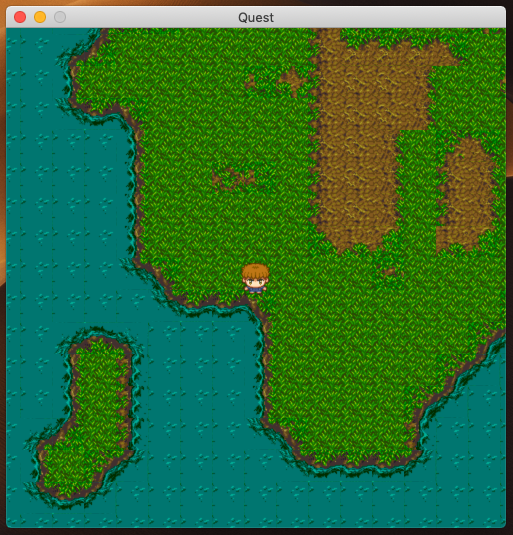
✅ CHECKPOINT:
Make sure everyone in your group got Quest working and was able to play the example games.
Exploring the Quest Framework
✏️
The rest of this lab will ask you to explore the Quest framework and write answers
to questions in lab_journal.md
. Don’t forget to commit and push your work.
⚡✨
Q0. Before you look at any code, make a prediction about what classes are being used in the island adventure game. For each class you imagine, describe:
- The name of the class
- What it does
- What other classes it interacts with
Q1. You may have noticed some of the following features. For each, explain how the classes you imagined in Q0 could make this happen. Extend your list of imagined classes if necessary.
- Q1a. This game is made up of a bunch of little images called sprites. The player is a sprite, and each patch of background is a sprite. How could the game keep track of which sprites belong where?
- Q1b. When you press the arrow keys, the player sprite moves around. Which class should receive these key-press events? What behavior might this class be doing when the arrow keys are pressed?
- Q1c. The player is not allowed to walk into the ocean. How could the game enforce this rule?
- Q1d. As the player moves around, the viewport scrolls, so that the player can never get to the edge of the screen. Which class could make this happen? What rule would be applied to get this behavior?
Now open island.py
and read the code for the game we just played.
First, skim the whole file. At the top there are a whole bunch of import statements importing Quest classes.
The rest of the module consists of a class called IslandAdventure
, and then
three lines at the end which create an instance of IslandAdventure
.
💻 Try changing some of IslandAdventure
’s values, saving the file, and running it again.
⚡✨
Q2. What does each of the following attributes do? What happens when you change them?
- Q2a. screen_width
- Q2b. top_viewport_margin
- Q2c. player_initial_x
- Q2d. player_speed
Class inheritance
Line 8 declares IslandAdventure
as a subclass of quest.game.QuestGame
:
|
|
When a class is a subclass of another class, it inherits all of the parent class’s attributes
and methods, along with any new attributes and methods defined in the child class. If the child
class defines an attribute or method which is also present in the parent, the child overrides
the parent method. In Quest, every game will be a subclass of QuestGame
, inheriting all the
default behavior and overriding methods as necessary to customize specific behaviors.
The built-in function super()
returns a class’s parent class. You will frequently see subclasses
using super()
to call a parent method within an overridden method. This allows a subclass to
perform all of behaviors in the parent method and then to add additional behaviors of its own.
👀
Skim at the source code for quest/game.py
.
The QuestGame
class has a bunch of methods like setup_maps()
, setup_walls()
,
setup_player()
, setup_npcs()
, and so on. Most of these do almost nothing.
The idea is that subclasses like IslandAdventure
can override these methods
when they need to.
⚡✨
Q3. Inisland.py
,IslandAdventure
overrides two methods from its parent class,QuestGame
. What are they? What do these methods do? One of these overridden methods callssuper()
. Why?
⚡✨
Q4. When you press the arrow keys (or wasd), the player moves around the island. How does this happen? Using the documentation forQuestGame
, explain which methods are involved.
💻 Now play a second version of Island Adventure by running python island_discrete.py
.
There is a very slight difference in the way the player moves.
⚡✨
Q5. Explain the difference, using the words “continuous” and “discrete” (look ’em up). Then explain howIslandAdventureDiscrete
achieves this. Which class actually determines whether movement is continuous or discrete? Finally, explain why a game might want to use discrete movement like this.
💻 Now play another sample game
by running python grandmas_soup.py
.
GrandmasSoup
shows how dialogue can be used in a Quest game. There are two main classes that help
manage dialogue: Modal
and Dialogue
.
⚡✨
Q6. Using the source code forgrandmas_soup.py
and the Quest documentation, explain the role ofModal
andDialogue
in the Grandmas’s Soup game.
Interactions in the game happen when the Player
collides with an NPC
. GrandmasSoupGame
extends the NPC
class into two new classes: Grandma
and Vegetable
(which itself has further subclasses like Carrot
).
⚡✨
Q7. What happens when the player collides with Grandma? Which methods are involved? What happens when the player collides with a vegetable? Which methods are involved?
💻 Play the last example game
by running python maze.py
.
Read the source code for the Maze
class. Note how much of it is comments.
💻 Make some sample mazes in a terminal window by opening the python shell and running the following code:
>>> from quest.maze import Maze
>>> m = Maze(55, 15)
>>> m.generate()
>>> print(m)
⚡✨
Q8. Describe, in your own words, how a depth-first maze is constructed.
Your turn
In this lab you explored how a few example games were written using Quest. Now, in the unit project, it’s your turn to implement a game of your own.