Lists and dicts
Lists
So far, every variable you have used has contained exactly one value. Data structures are containers which allow you to keep track of multiple values.
Lists are pretty simple data structures. They store a bunch of items in a sequence. Think for a moment about the ways you might want to interact with a list:
- Create a list by describing what should be in it.
- Add an item to the beginning. Or to the end. Or in the middle.
- Get the third item from the list. Or the tenth. Or the second-to-last.
- Check if something is in the list.
- Check how many items the list contains.
๐ป Open a Python shell and try these out yourself. Seriously, do this. Don’t just read it over.
>>> colors = ["red", "green", "blue"] # create a list
>>> colors
["red", "green", "blue"]
>>> colors.append("orange") # add an item to the end
>>> colors
["red", "green", "blue", "orange"]
>>> colors.insert(0, "white") # add an item at a position
>>> colors
["white", "red", "green", "blue", "orange"]
>>> colors[0] # get an item
"white"
>>> colors[2]
"green"
>>> colors[-1] # counting back from the end
"orange"
>>> "yellow" in colors # check if an item is included
False
>>> len(colors) # check the list's length
5
>>> for color in colors: # iterate over the list
print(color + " is a fine color.")
white is a fine color.
red is a fine color.
green is a fine color.
blue is a fine color.
orange is a fine color.
๐พ ๐ฌ
To iterate means to do something repeatedly. In Python, we often talk about iterating over a list, which means going through the list one item at a time using a for loop. You already learned how to iterate over ranges, using
for number in range(10)
. Arange
is different from a list, but it has the same structure–a sequence of items, one after the other. Whenever something has this structure, we call it an iterable and we can generally interact with it like a list.Consider another iterable you already know: strings. A string is just a sequence of characters, so it won’t be a surprise that you can do the following:
>>> word = "incomprehensible" >>> len(word) 16 >>> word[2] "c" >>> "y" in word False
Functional programming with lists
As a reminder, functional programming is a way of solving problems by thinking about how inputs get transformed into outputs. Functional programming is deeply connected to lists–the original functional programming language was called lisp, short for “list processing.”
Now, we will utilize a functional programming approach to transform lists.
list_functions.py
contains the following four functions. Your job is to
finsh them.
square_lists()
capitalize_list()
pluralize_list()
reverse_list()
Each function takes a list as a parameter, and returns and new list.
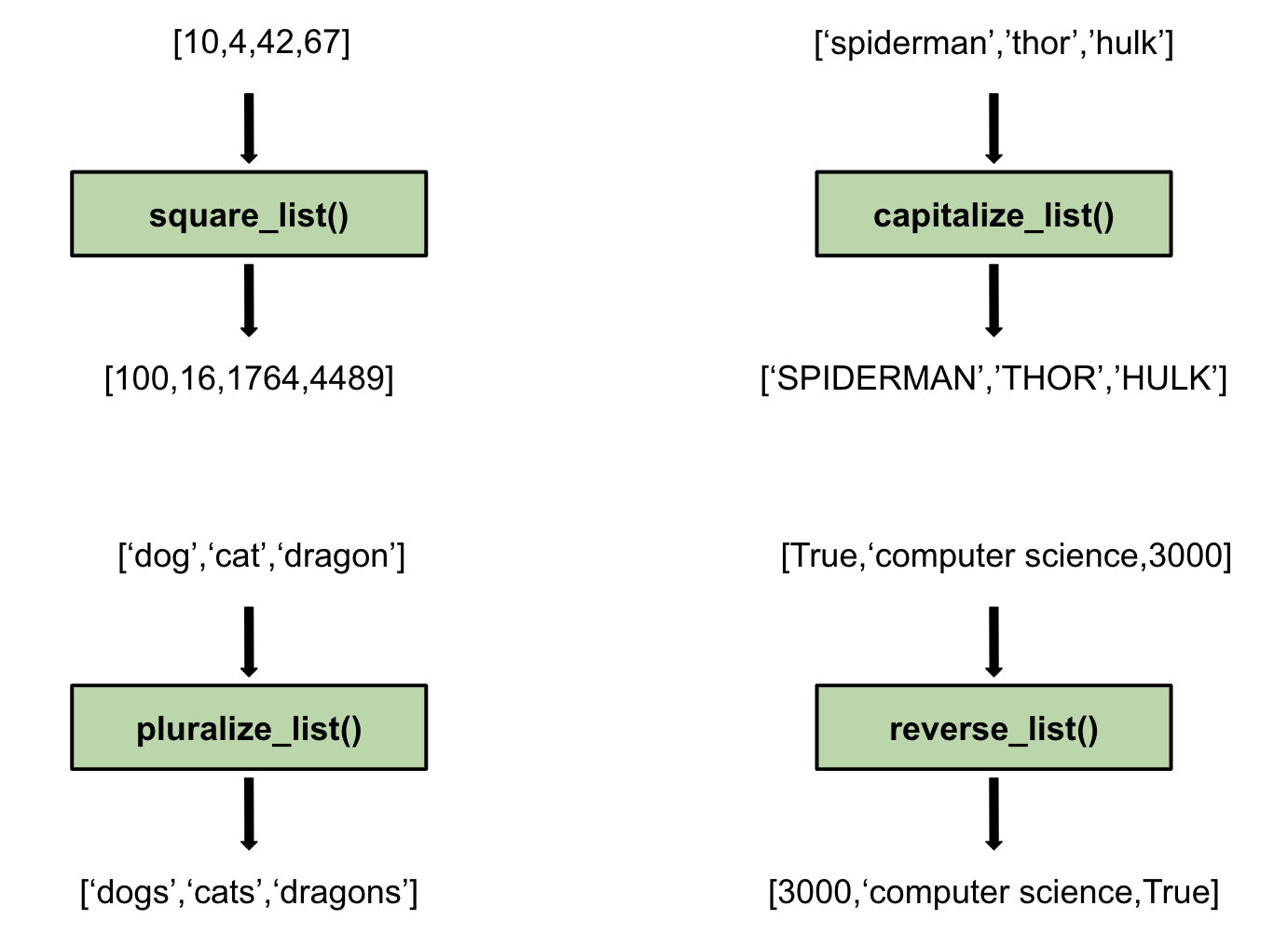
๐ป Code the function
square_list()
- Parameter: a list of numbers
- Return value: a new list with each element squared
๐ป Code the function
capitalize_list()
- Parameter: a list of strings
- Return Value:a new list with each string fully capitalized
๐ป Code the function
pluralize_list()
- Parameter: a list of strings
- Return Value: a new list word in its plural form
๐ป Code the function
reverse_list()
- Parameter: Takes a list of items (can be any data type)
- Return Value: a new list with items in reverse order
Here are a few helpful functions to transform the elements in the lists.
Function | Data Type | Explanation | Example |
---|---|---|---|
append(element ) |
lists | adds an element to the end of a list | my_list.append("lemonade") |
upper() |
strings | capitalizes every letter in a string | my_string.upper() |
Testing
The best way to check that a program is working correctly is… with another program!
This lab contains the module test_list_functions.py
, which will import your list functions
and test them. Try running them now–we expect them to fail at first.
$ python test_list_functions.py
Re-run these tests whenever you finish a function to make sure it’s correct.
โ CHECKPOINT:
Answer the following prompts in your notebook:
- What is a list and why is it useful?
- What are the benefits of using a functional programming approach to list transformations?
- Can you think of situations where software testing would help prevent everyday problems?
โกโจ
Save, commit, and push your work after you finish each function–it’s a good idea to get in the habit of committing every time you finish a meaningful group of changes to your code.
Dictionaries
Whereas lists hold items in a sequence numbered by integers (item 0, item 1, item 2, …), dictionaries hold items in a key-value structure (also called a mapping). Each key in a dictionary is like the label on a drawer; each value is like the contents of the drawer. The key should be a string; the value can be just about anything, including another list or dictionary. Here is an example of a dictionary.
>>> country_land_area = {
"Russia": 17098242,
"Canada": 9984670,
"China": 9706961,
"USA": 9372610,
"Brazil": 8515767,
}
>>> country_land_area["Canada"]
9984670
>>> "India" in country_land_area
False
>>> country_land_area["Australia"] = 7692024
Each number corresponds to a country. And you can look up a country’s area (in square kilometers) using its name. The structure of this data is well-suited to using a dictionary. You could store it in a list of lists, as shown below, but it would be much clumsier to work with.
>>> country_land_area = [
["Russia", 17098242],
["Canada", 9984670],
]
To look up Canada’s area, you would have to go through the list, looking at the first item of each sub-list until you found “Canada”, and then take the second item of that list. And then you’d need to deal with the possibility that Canada’s area is not listed. Choosing the right data structure for a problem can have a big impact on how easy or difficult it is to solve the problem.
List or dict?
โ๏ธ
Open list_or_dict.md
in this lab’s directory. For each use case below, explain whether
it would be better to store the data in a list
or in a dict
. (Not all of these have a clear answer, so justify
yours.)
- Items you need to buy at the grocery store
- Your friends’ birthdays
- Keeping track of RSVPs to a party
- Your personal movie ratings
- Temperature readings from a sensor
- Messages in an email inbox
- Git commits
โกโจ
When you finish, push your work to the server.
Iterating over dictionaries
As with lists, we sometimes need to go through all the the contents of a dictionary, one by one. There are three main ways to do this:
>>> for key in country_land_area.keys():
print(key)
Russia
Canada
China
USA
Brazil
>>> for value in country_land_area.values():
print(value)
17098242
9984670
9706961
9372610
8515767
>>> for key, value in country_land_area.items():
print("The area of {} is {}.".format(key, value))
The area of Russia is 17098242.
The area of Canada is 9984670.
The area of China is 9706961.
The area of USA is 9372610.
The area of Brazil is 8515767.