Conditionals
In this lab, we’ll introduce a new concept that will allow us to make even more interesting drawings: conditionals.
Conditional behavior
One of the most powerful ways to utilize computation is to change the behavior of our code based on context. This might sound complicated, but as a human you are very familiar with this kind of response. There are many situations where you change your behavior based on the environment. For example, if it is raining outside, you wear rain boots. Otherwise, you might wear a different kind of shoe like tennis shoes. You probably have even more complicated environmental responses as well. For example, if you are ordering bubble tea, your order might go like this:
- If the shop has taro,
- you get taro in your tea
- If the shop doesn’t have taro but has pearl
- you get pearl in your tea
- Finally, in the case that the shop doesn’t have taro or pearl
- you don’t order any bubble tea.
In computer science, we call this kind of behavior conditional: some parts of your code only run if a condition is satisfied. No taro and no pearl in the shop? Then you’re not buying tea.
👾 💬 The Python shell
In the exercise below you are going to try out short pieces of Python code. You could do this the usual way, opening a file in Atom, saving it, and then running it in Terminal. But there’s a simpler way: you can enter a Python shell where you see the result of each line you type right away.
To enter a Python shell, just type
python3
into your terminal (or justpython
if you’re inside a poetry shell). Use theexit()
command orControl+D
to close the Python shell. A Python shell executes Python line-by-line. It’s useful for testing out code, but usually you’ll write programs in separate files where you can save your work. Note that the Python shell prompt,>>>
is different from the regular terminal prompt.
Conditions
In computer science, conditions are binary. They are either True
or False
, never in-between.
You can use comparison operators to write expressions which evaluate to True
or False
.
Predict the value of each of the following expressions.
2 < 3
2 == 3
2 != 3
(2 + 4 > 10) or (3 + 3 == 6)
(2 + 4 > 10) and (3 + 3 == 6)
True or False
True and False
(True or False) and (True or True)
not False
not (2 > 3 or 3 > 4 or 4 > 5)
✅ CHECKPOINT:
Using the Python shell to experiment, fill out the chart below in your notebook.
You can use the following operators to compare numbers:
<
,>
,==
,<=
,>=
,!=
. The resulting expression will evaluate to a boolean value,True
orFalse
. You can use the operatorsand
,or
, andnot
to write expressions with booleans.Be sure to define what each of the operators does and give an example of when the operator will generate a
True
condition and when the operator will generate aFalse
condition:
Operator Description True example False example <
>
==
!=
<=
>=
and
or
not
👾 💬
Make sure you understand the difference between=
and==
. This is one of the biggest sources of confusion for beginner Pythonistas.=
is used for assigning a variable, whereas==
is for checking whether two things are equal.
Conditionals
Using the conditions generated by comparison operators, you can conditionally execute pieces of your code. This is useful for changing what your code does to respond to different conditions of the program.
if statements
if
statements are the beginning to every conditional code block. The code written inside the code block that follows only runs if the condition after the if
evaluates to True
.
for i in range(20):
if i < 10:
print(i, " is smaller than 10")
else statements
else
statements can be paired with if
statements to create an alternative block of code to execute if the condition after the if
evaluates to False
.
What is the difference between the following two programs?
# [else: example 1]
for i in range(20):
if i < 10:
print(i, " is smaller than 10")
print(i, " is greater than or equal to 10")
# [else: example 2]
for i in range(20):
if i < 10:
print(i, " is smaller than 10")
else:
print(i, " is greater than or equal to 10")
✅ CHECKPOINT:
In your notebook, write what each example will output.
elif statements
Finally, elif
statements (“else if”) can be used to create multiple branches of a conditional. These statements add another condition to check if the condition above them does not pass.
The following program creates three branches of execution:
for i in range(20):
if i < 10:
print(i, " is smaller than 10")
elif i < 15:
print(i, " is greater than 9 but less than 15")
else:
print(i, " is greater than or equal to 15")
This conditional creates the folloing cases for the variable i
:
- i < 10
- 10 <= i < 15
- 15 <= i
Conditionals: User Input
Navigate to making_with_code/pedprog/unit00/lab04
and enter a poetry shell. (As always, if this directory doesn’t exist, run mwc update
.)
Now open conditionals_user_input.py
in your text editor. Read this program and predict what it will do.
|
|
💻 Now run the program. Read the code again and make sure you understand the meaning of every line. This program has a lot of potential, but so far it can only generate one drawing.
💻 Add at least two more branches to the conditional so that the program can draw more shapes.
An elif
statement will probably be useful here.
✅ CHECKPOINT:
Answer the following check-in questions in your notebook before moving on:
- What is the difference between
else
andelif
statements in a conditional?- What is the difference between
=
and==
?- When writing a conditional, what kinds of things can you compare? Can you compare different types of things to each other?
Modulo
Python has many operators that allow you to perform calculations with values. You’ve probably
seen and used the basic ones like +
(add), -
(subtract), *
(multiply), and /
(divide).
However, Python has some other less common opertors that can be really helpful.
One such operator is the modulo operator (%
). This operator takes two values, divides them, and returns the remainder of the division.
✅ CHECKPOINT:
Using the Python shell to experiment, fill out the chart below in your notebook.
Calculation Result 0 % 3
1 % 3
2 % 2
3 % 6
4 % 0
5 % 2
6 % 6
7 % 0
Rainbow
Conditionals can also be paired with the modulo operator to cause your code to run in repeated
patterns. Open conditionals_modulo.py
read the program, and run it to get a sense of how it works.
|
|
This code is really long. However, there is a pattern to the rainbow that we could use to simplify the code: the rainbow has 7 colors that repeat every time the loop runs for 5 iterations.
💻 Simplify this code using a conditional and the modulo operator. Your program should be less than 40 lines of code.
✅ CHECKPOINT:
Answer the following questions in your journal:
- How would you describe the modulo operator to a friend who has never heard of it before?
- Describe a moment when your code didn’t do what you intended. How did you fix it?
Extension
Coloring Fibonacci
You’ve seen a great example of modulo being used to select the color of a drawing. Now you can use your newfound knowledge!
💻 Incorporate the modulo operator into your fibonacci visualization from the previous lab. If you didn’t get a change to create a visualization of the fibonacci sequence last class, now is your opportunity!
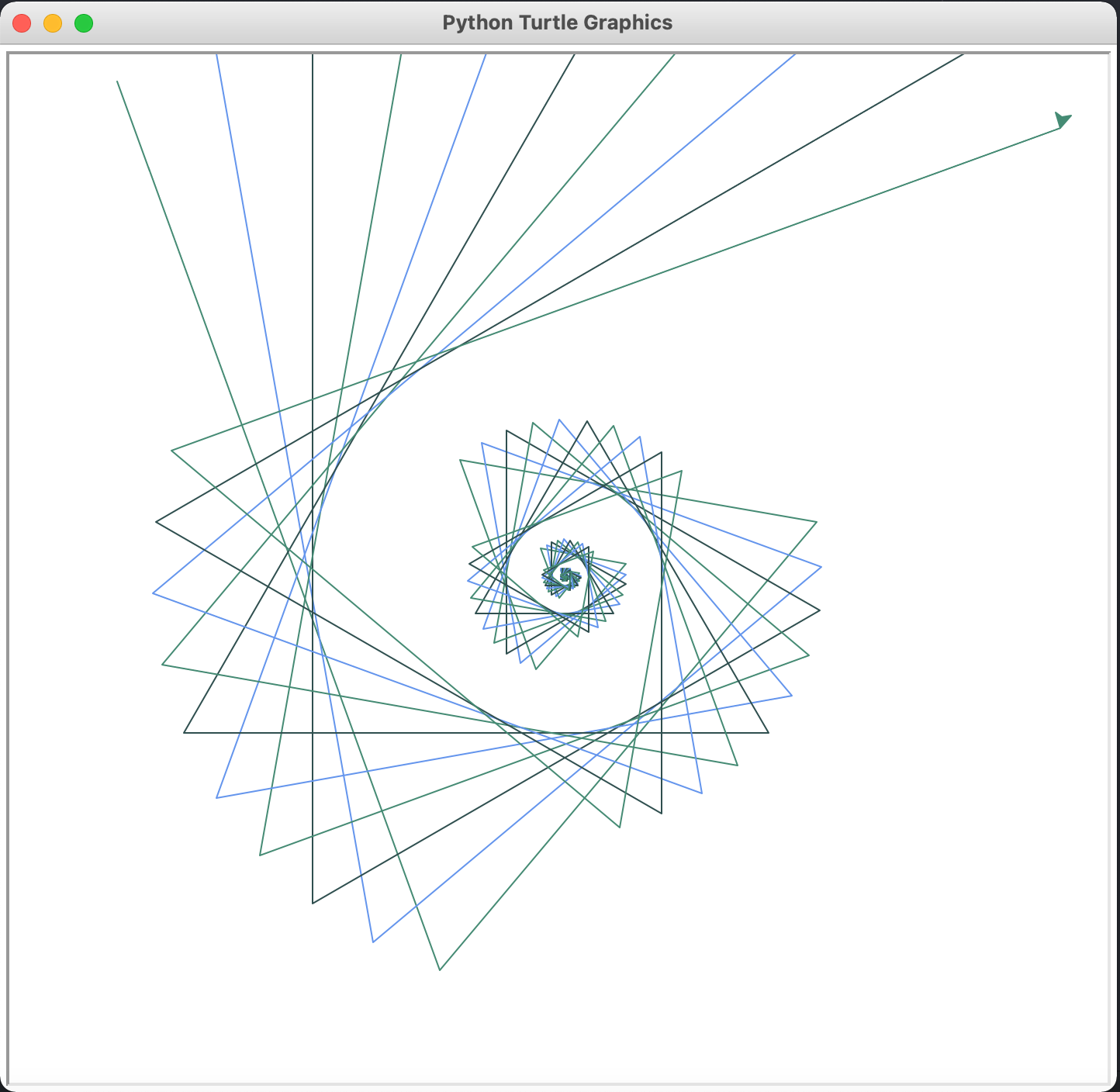